Demographic Use Cases
New Hire
When creating a new hire using PlanSource's API, you will need to utilize the POST /subscriber endpoint. This endpoint allows the developer to create a new subscriber resource (new employee). There are many subscriber data fields within that endpoint, but not all of them are required to create a new employee. To see a full list of the subscriber data fields, visit the Table of Subscriber Fields. From that table, only a few fields are required to create a new subscriber.
Required fields can be managed/changed in PlanSource's Benefits Administration site under Data Field Security.
You can see an example of regularly required fields below along with its corresponding API call:
Data Fields | Field Descriptions |
---|---|
ssn | SSN |
first_name | First Name |
last_name | Last Name |
address_1 | Address 1 |
city | City |
state | State |
zip_code | Zip |
gender | Gender |
marital_status | Marital Status |
employment_level | Employment Level |
hire_date | Hire Date |
birthdate | Birth Date |
Code:
curl --request POST \
--url https://example.com/admin/subscriber \
--data '{"ssn":"123-456-7891","first_name":"John","last_name":"Smith","address_1":"1234 Highway Ln","city":"Orlando","state":"FL",
"zip_code":"34786","gender":"Male","marital_status":"Married","employment_level":"FT","hire_date":"04/12/2018","birthdate":"01/01/1994"}'
var request = require("request");
var options = { method: 'POST',
url: 'https://example.com/admin/subscriber',
body:
{ ssn: '123-456-7891',
first_name: 'John',
last_name: 'Smith',
address_1: '1234 Highway Ln',
city: 'Orlando',
state: 'FL',
zip_code: '34786',
gender: 'Male',
marital_status: 'Married',
hire_date: '04/12/2018',
birthdate: '01/01/1994' },
json: true };
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
require 'uri'
require 'net/http'
url = URI("https://example.com/admin/subscriber")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request.body = "{\"ssn\":\"123-456-7891\",\"first_name\":\"John\",\"last_name\":\"Smith\",\"address_1\":\"1234 Highway Ln\",\"city\":\"Orlando\",
\"state\":\"FL\",\"zip_code\":\"34786\",\"gender\":\"Male\",\"marital_status\":\"Married\",\"hire_date\":\"04/12/2018\",\"birthdate\":\"01/01/1994\"}"
response = http.request(request)
puts response.read_body
var data = JSON.stringify({
"ssn": "123-456-7891",
"first_name": "John",
"last_name": "Smith",
"address_1": "1234 Highway Ln",
"city": "Orlando",
"state": "FL",
"zip_code": "34786",
"gender": "Male",
"marital_status": "Married",
"hire_date": "04/12/2018",
"birthdate": "01/01/1994"
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://example.com/admin/subscriber");
xhr.send(data);
import requests
url = "https://example.com/admin/subscriber"
payload = "{\"ssn\":\"123-456-7891\",\"first_name\":\"John\",\"last_name\":\"Smith\",\"address_1\":\"1234 Highway Ln\",\"city\":\"Orlando\",\"state\":
\"FL\",\"zip_code\":\"34786\",\"gender\":\"Male\",\"marital_status\":\"Married\",\"hire_date\":\"04/12/2018\",\"birthdate\":\"01/01/1994\"}"
response = requests.request("POST", url, data=payload)
print(response.text)
Result:
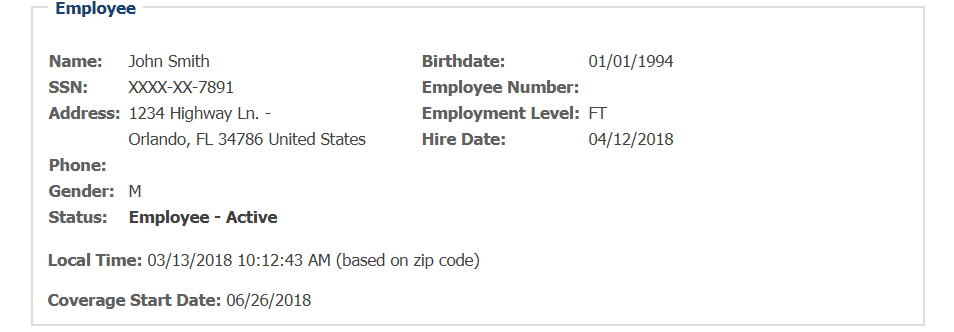
Update
When updating an existing subscriber using PlanSource's API, you will need to utilize the PUT /subscriber/{id} endpoint. This endpoint allows the developer to update an existing subscriber resource with any new information that needs to be added. There are many subscriber data fields within the endpoint. To see a full list of the subscriber data fields, visit the Table of Subscriber Fields.
Required fields are defined by the Data Field Security.
From the list of Subscriber Fields, the developer can choose which data fields they would like to update. The only required data field that must be filled in is the id field. This field corresponds to the existing subscriber the developer would like to update.
The subscriber's unique ID can be configured manually by the developer when first creating the subscriber (setting the data field is_custom_id to True and inputting an ID to the data field subscriber_code) or the ID can be configured automatically by the PlanSource system when the subscriber first gets created (setting the data field is_custom_id to False and the ID data field automatically populates when the subscriber is created).
You can see an example of some fields that are used to update a subscriber below in the API call:
Code:
curl --request PUT \
--url https://example.com/admin/subscriber/87459963 \
--data '{"address_1":"281 Tysons Corner","city":"Mclean","state":"Virginia","zip_code":"37845","employment_level":"PT"}'
var request = require("request");
var options = { method: 'PUT',
url: 'https://example.com/admin/subscriber/87459963',
body:
{ address_1: '281 Tysons Corner',
city: 'Mclean',
state: 'Virginia',
zip_code: '37845',
employment_level: 'PT' },
json: true };
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
require 'uri'
require 'net/http'
url = URI("https://example.com/admin/subscriber/87459963")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Put.new(url)
request.body = "{\"address_1\":\"281 Tysons Corner\",\"city\":\"Mclean\",\"state\":\"Virginia\",\"zip_code\":\"37845\",\"employment_level\":\"PT\"}"
response = http.request(request)
puts response.read_body
var data = JSON.stringify({
"address_1": "281 Tysons Corner",
"city": "Mclean",
"state": "Virginia",
"zip_code": "37845",
"employment_level": "PT"
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("PUT", "https://example.com/admin/subscriber/87459963");
xhr.send(data);
import requests
url = "https://example.com/admin/subscriber/87459963"
payload = "{\"address_1\":\"281 Tysons Corner\",\"city\":\"Mclean\",\"state\":\"Virginia\",\"zip_code\":\"37845\",\"employment_level\":\"PT\"}"
response = requests.request("PUT", url, data=payload)
print(response.text)
Result:
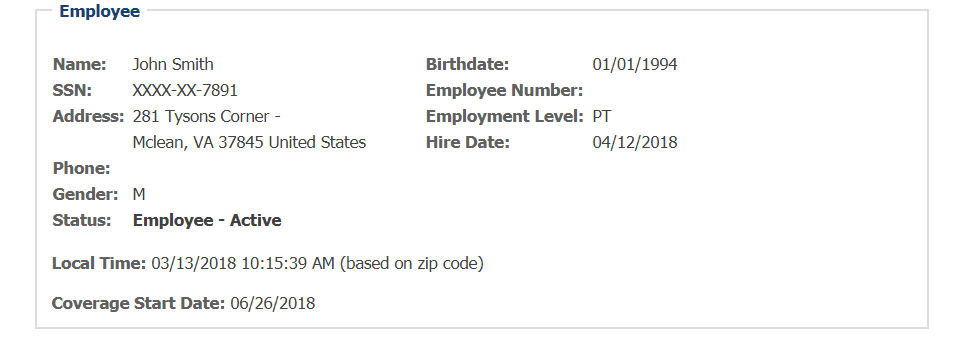
Terminate
When terminating an existing subscriber using PlanSource's API, you will need to utilize the PUT /subscriber/{id}/terminate endpoint. This endpoint allows the developer to terminate an existing subscriber resource without deleting them completely from the system.
There are four main data fields that can be filled out to terminate the subscriber:
- ID (the unique ID corresponding to the existing subscriber) Required
- termination_reason (the reason for terminating the subscriber) Required
- termination_date (the subscriber's last day / termination date) Required
- voluntary (a true or false value for whether the termination was voluntary or not)
termination_reason is a list validated field. The developer first needs to call a meta endpoint to receive all of the available termination reasons. Once the list comes back, the developer can choose and input a reason for the required field.
See Guide on List Validated Fields for more info.
See Meta Endpoints for more info.
Below is a list of standard termination reasons as an example.
COBRA Termination Reasons | Non-COBRA Termination Reasons |
---|---|
Termination of Employment | Termination of Employment for gross misconduct |
Retirement | Other Non-COBRA Employment Termination |
Death | Terminate Existing COBRA coverage |
Terminated due to change in employment level | |
Termed due to LOA | |
Disability |
You can see an example of the fields that are used to terminate a subscriber below in the API call:
Code:
curl --request PUT \
--url https://example.com/admin/subscriber/87459963/terminate \
--data '{"termination_reason":"employment_termination_for_cause","termination_date":"4/13/2018","volunatary":"false"}'
var request = require("request");
var options = { method: 'PUT',
url: 'https://example.com/admin/subscriber/87459963/terminate',
body:
{ termination_reason: 'Termination of Employment for gross misconduct (Non-COBRA)',
termination_date: '4/13/2018',
volunatary: 'false' },
json: true };
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
require 'uri'
require 'net/http'
url = URI("https://example.com/admin/subscriber/87459963/terminate")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Put.new(url)
request.body = "{\"termination_reason\":\"Termination of Employment for gross misconduct (Non-COBRA)\",\"termination_date\":\"4/13/2018\",
\"volunatary\":\"false\"}"
response = http.request(request)
puts response.read_body
var data = JSON.stringify({
"termination_reason": "Termination of Employment for gross misconduct (Non-COBRA)",
"termination_date": "4/13/2018",
"volunatary": "false"
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("PUT", "https://example.com/admin/subscriber/87459963/terminate");
xhr.send(data);
import requests
url = "https://example.com/admin/subscriber/87459963/terminate"
payload = "{\"termination_reason\":\"Termination of Employment for gross misconduct (Non-COBRA)\",\"termination_date\":\"4/13/2018\",
\"volunatary\":\"false\"}"
response = requests.request("PUT", url, data=payload)
print(response.text)
Result:
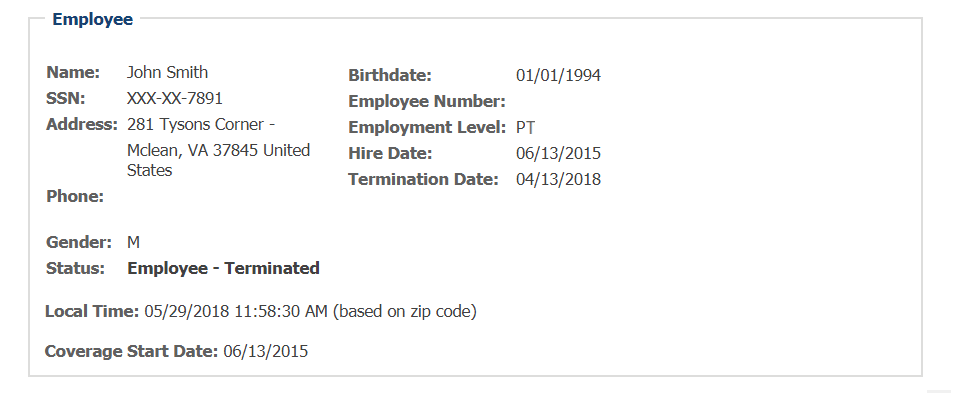
Rehire
When rehiring a terminated subscriber using PlanSource's API, you will need to utilize the PUT /subscriber/{id}/rehire endpoint. This endpoint allows the developer to rehire a terminated subscriber resource.
There are two main data fields that can be filled out to rehire the subscriber:
- ID (the unique ID corresponding to the terminated subscriber) Required
- rehire_date (the date on which the terminated subscriber will be rehired) Required
You can see an example of the fields that are used to rehire a terminated subscriber below in the API call:
Code:
curl --request PUT \
--url https://example.com/admin/subscriber/87459963/rehire \
--data '{"rehire_date":"04/14/2018"}'
var request = require("request");
var options = { method: 'PUT',
url: 'https://example.com/admin/subscriber/87459963/rehire',
body: { rehire_date: '04/14/2018' },
json: true };
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
require 'uri'
require 'net/http'
url = URI("https://example.com/admin/subscriber/87459963/rehire")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Put.new(url)
request.body = "{\"rehire_date\":\"04/14/2018\"}"
response = http.request(request)
puts response.read_body
var data = JSON.stringify({
"rehire_date": "04/14/2018"
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("PUT", "https://example.com/admin/subscriber/87459963/rehire");
xhr.send(data);
import requests
url = "https://example.com/admin/subscriber/87459963/rehire"
payload = "{\"rehire_date\":\"04/14/2018\"}"
response = requests.request("PUT", url, data=payload)
print(response.text)
Result:
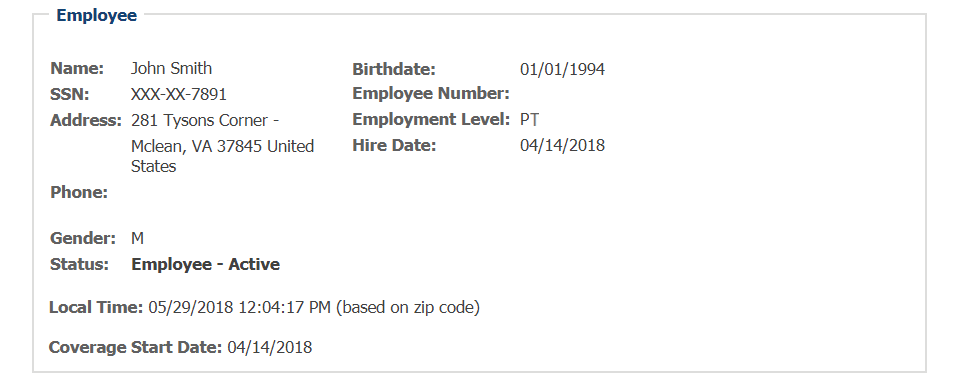
Updated over 5 years ago